前言
随着SpringBoot
的流行,我们已经很少会单独拿一个框架出来使用,大部分都会经过starter
的包装来使我们对于框架的集成和使用很方便。但是,万变不离其宗,掌握最原始的使用方法对我们对于框架的掌握和使用还是很有帮助的。
本博文主要是记录单独使用MyBatis
框架时,配置文件的一些详细配置项,以作记录。
配置文件详解
核心配置文件
总览
MyBatis
的核心配置文件的层级结构如下图所示:
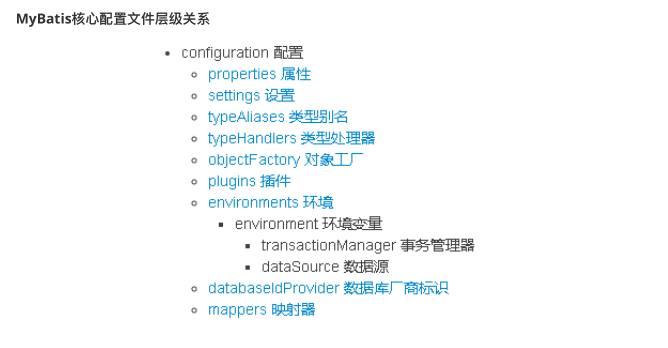
下面,我们对主要的配置项展开详细介绍。
environments标签
数据库环境的配置,支持多环境配置,细节如下:

其中,事务管理器(transactionManager
)类型有两种:
- JDBC:这个配置就是直接使用了
JDBC
的提交和回滚设置,它依赖于从数据源得到的连接来管理事务作用域。 - MANAGED:这个配置几乎没做什么。它从来不提交或回滚一个连接,而是让容器来管理事务的整个生命周期(比如 JEE 应用服务器的上下文)。 默认情况下它会关闭连接,然而一些容器并不希望这样,因此需要将
closeConnection
属性设置为 false 来阻止它默认的关闭行为。
其中,数据源(dataSource
)类型有三种:
- UNPOOLED:这个数据源的实现只是每次被请求时打开和关闭连接。
- POOLED:这种数据源的实现利用“池”的概念将
JDBC
连接对象组织起来。 - JNDI:这个数据源的实现是为了能在如 EJB 或应用服务器这类容器中使用,容器可以集中或在外部配置数据源,然后放置一个 JNDI 上下文的引用。
mapper标签
该标签的作用是加载映射的,加载方式有如下几种:
- 使用相对于类路径的资源引用,例如:
<mapper resource="org/mybatis/builder/AuthorMapper.xml"/>
- 使用完全限定资源定位符(URL),例如:
<mapper url="file:///var/mappers/AuthorMapper.xml"/>
- 使用映射器接口实现类的完全限定类名,例如:
<mapper class="org.mybatis.builder.AuthorMapper"/>
(注意:保证Mapper接口和xml的包路径一样,也就是说com.rubin.mapper.UserMapper对应的xml路径应该是classpath:com/rubin/mapper/UserMapper.xml) - 将包内的映射器接口实现全部注册为映射器,例如:
<package name="org.mybatis.builder"/>
(注意:保证Mapper接口和xml的包路径一样,也就是说com.rubin.mapper.UserMapper对应的xml路径应该是classpath:com/rubin/mapper/UserMapper.xml)
Properties标签
我们在实际开发中,习惯将数据源的配置信息单独抽取成一个properties
文件,properties
标签就可以加载额外配置的properties
文件。具体使用方式如下:

typeAliases标签
类型别名是为Java
类型设置一个短的名字。比如说原来的类型名称配置如下:

配置typeAliases
,为com.lagou.domain.User
定义别名为user
,如下图所示:

上面我们是自定义的别名,MyBatis
框架已经为我们设置好的一些常用的类型的别名,如下图:
映射配置文件mapper.xml
动态SQL之条件判断
动态SQL
比较好理解,就是根据条件的变化,我们的SQL
也会变化来适应业务。示例如下所示:
<select id="findByCondition" parameterType="user" resultType="user">
select * from User
<where>
<if test="id!=0">
and id=#{id}
</if>
<if test="username!=null">
and username=#{username}
</if>
</where>
</select>
当查询条件id
和username
都存在时,控制台打印的sql语句如下:
… … …
//获得MyBatis框架生成的UserMapper接口的实现类
UserMapper userMapper = sqlSession.getMapper(UserMapper.class);
User condition = new User();
condition.setId(1);
condition.setUsername("lucy");
User user = userMapper.findByCondition(condition);
… … …

当查询条件只有id存在时,控制台打印的sql语句如下:
… … …
//获得MyBatis框架生成的UserMapper接口的实现类
UserMapper userMapper = sqlSession.getMapper(UserMapper.class);
User condition = new User();
condition.setId(1);
User user = userMapper.findByCondition(condition);
… … …

动态SQL之条件循环
循环执行SQL
的拼接操作是将我们的集合参数为基础,遍历集合来拼装SQL
。例如:SELECT * FROM USER WHERE id IN (1,2,5)
。
<select id="findByIds" parameterType="list" resultType="user">
select * from User
<where>
<foreach collection="list" open="id in(" close=")" item="id" separator=",">
#{id}
</foreach>
</where>
</select>
测试代码片段以及控制台SQL打印如下:
… … …
//获得MyBatis框架生成的UserMapper接口的实现类
UserMapper userMapper = sqlSession.getMapper(UserMapper.class);
int[] ids = new int[]{2,5};
List<User> userList = userMapper.findByIds(ids);
System.out.println(userList);
… … …

foreach
标签的属性含义如下:
- collection:代表要遍历的集合元素,注意编写时不要写#{}。
- open:代表语句的开始部分。
- close:代表结束部分。
- item:代表遍历集合的每个元素,生成的变量名。
- sperator:代表分隔符。
SQL片段抽取
SQL
片段去抽也就是将SQL
中重复的SQL
提取出来,使用时用 include
引用即可,最终达到SQL
重用的目的。示例如下:
<!--抽取sql片段简化编写-->
<sql id="selectUser" select * from User</sql>
<select id="findById" parameterType="int" resultType="user">
<include refid="selectUser"></include> where id=#{id}
</select>
<select id="findByIds" parameterType="list" resultType="user">
<include refid="selectUser"></include>
<where>
<foreach collection="array" open="id in(" close=")" item="id"separator=",">
#{id}
</foreach>
</where>
</select>
文章评论